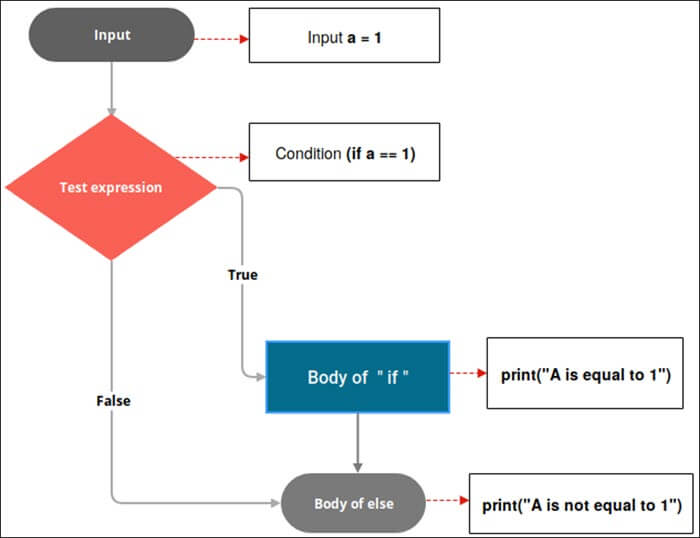
Welcome to day 8 & 9 the next phase of your Python learning journey! Today, we dive deeper into the realm of conditional statements (if, else, else if) and comparison operators. Understanding these concepts is fundamental to creating dynamic and responsive programs. So, let’s explore how they work and how you can leverage them in your Python code.
Conditional Statements in Python
if Statement: The if
statement is the cornerstone of conditional logic. It allows your program to make decisions based on whether a specified condition is true or false. Here’s a basic example:
# Example 1: Simple if statement
age = 25
if age < 18:
print("You are a minor.")
In this example, the program checks if the variable age
is less than 18. If the condition is true, the indented block of code under the if
statement is executed.
else Statement: The else
statement complements the if
statement by providing an alternative action to be taken when the initial condition is false:
# Example 2: if-else statement
age = 25
if age < 18:
print("You are a minor.")
else:
print("You are an adult.")
If the condition in the if
statement is false, the block of code under else
is executed.
elif Statement: When you have multiple conditions to check, the elif
(else if) statement comes into play. It allows you to check additional conditions if the previous ones are false:
# Example 3: if-elif-else statement
score = 75
if score >= 90:
print("Excellent!")
elif 70 <= score < 90:
print("Good job!")
else:
print("Keep practicing.")
Here, the code evaluates different score ranges and prints a message accordingly.
Leveraging Comparison Operators
Comparison operators play a crucial role in conditional statements as they enable you to compare values. Here are some commonly used comparison operators:
==
: Equal to!=
: Not equal to<
: Less than>
: Greater than<=
: Less than or equal to>=
: Greater than or equal to
Practical Exercise
Let’s put your newfound knowledge to the test with a practical exercise:
# Exercise: Grade Classifier
# Ask the user to input a score, and classify it into different grade categories.
score = int(input("Enter the student's score: "))
if score >= 90:
grade = "A"
elif 80 <= score < 90:
grade = "B"
elif 70 <= score < 80:
grade = "C"
elif 60 <= score < 70:
grade = "D"
else:
grade = "F"
print(f"The student's grade is: {grade}")
This exercise prompts the user to input a score and classifies it into different grade categories using conditional statements and comparison operators.
Conclusion
Congratulations on delving into the intricacies of conditional statements and comparison operators in Python! These concepts are powerful tools that empower you to create dynamic and decision-making programs. As you move forward, continue to experiment with different conditions and scenarios to strengthen your understanding. Happy coding!
1 thought on “Understanding Python Conditional Statements and Comparison Operators”
Comments are closed.